For one of my educational project, a small electronic jewelry-like device meant to teach kids how to program through an artistic platform, we needed a way to present programs as stories that are pleasant to read. The Literate Programming style fulfills these requirements perfectly. Moreover, literate programming is a very natural paradigm for Arduino programs, as they are frequently built with a single code file. In such cases, a literate style is indispensable for keeping the code well organized and pedagogically understandable.
Pedagogical concerns also dictated that the literate program will need to be
rendered as a beautiful and easily accessible web page as well. For simplicity,
any such renditions would have to be derived from a single C++
source file
and its inline comments. Moreover, any markup used in these comments needs to
be pleasant to read from the Arduino IDE when students try to compile a given
sketch. This naturally led us to use Markdown for the comments.
The final result can be seen on one of our documentation pages, showcasing an entire library written in a literate style, with graphs and videos in between the code. It is a responsive design where the code and text span two separate columns only if the screen is wide enough. The style of this particular code file varies between literate and more classical commentary, which is natural for a library file. We plan to employ the literate style more heavily in the example sketches for our device.
The first step in obtaining this would be to transform code looking like this
// this is a comment
// a long comment
int a = some_function(b);
c = 3*a;
into the following markdown file (to be compiled by pandoc
so that the code
and markdown comments are rendered neatly)
<div class="comment">
this is a comment
a long comment
</div>
<div class="code">
```c++
int a = some_function(b);
c = 3*a;
```
</div>
The following short python
script does exactly this, by reading a
cpp
file and outputting a Markdown or HTML file with the rendition of the
literate program.
# Read the lines of the code file to be rendered
lines = open(INPUT_CODE_FILE).readlines()
def classify_line(line):
'''Given how the line starts, classify it as code or commentary.'''
if line.startswith('//'):
return 'comment'
if ...
# a few other types of lines
else:
return 'code'
output = [] # Store all of the lines of the output Markdown file in this list.
output.append('<div class="flex-container">')
for line_type, group_of_lines in itertools.groupby(lines, classify_line):
# Separate blocks of consecutive code lines from blocks
# of consecutive commentary lines and put each block
# in a div tag of a corresponding class.
output.append('<div class="%s">\n'%line_type)
output.append(''.join(group_of_lines))
output.append('</div>\n')
output.append('</div>')
with open(OUTPUT_FILE,'w') as f:
f.writelines(output)
With some minimal use of flexbox CSS the consecutive blocks of comments and
code can be rendered neatly in two responsive columns.
All of this can then simply be piped through pandoc
and your literate rendition is ready:
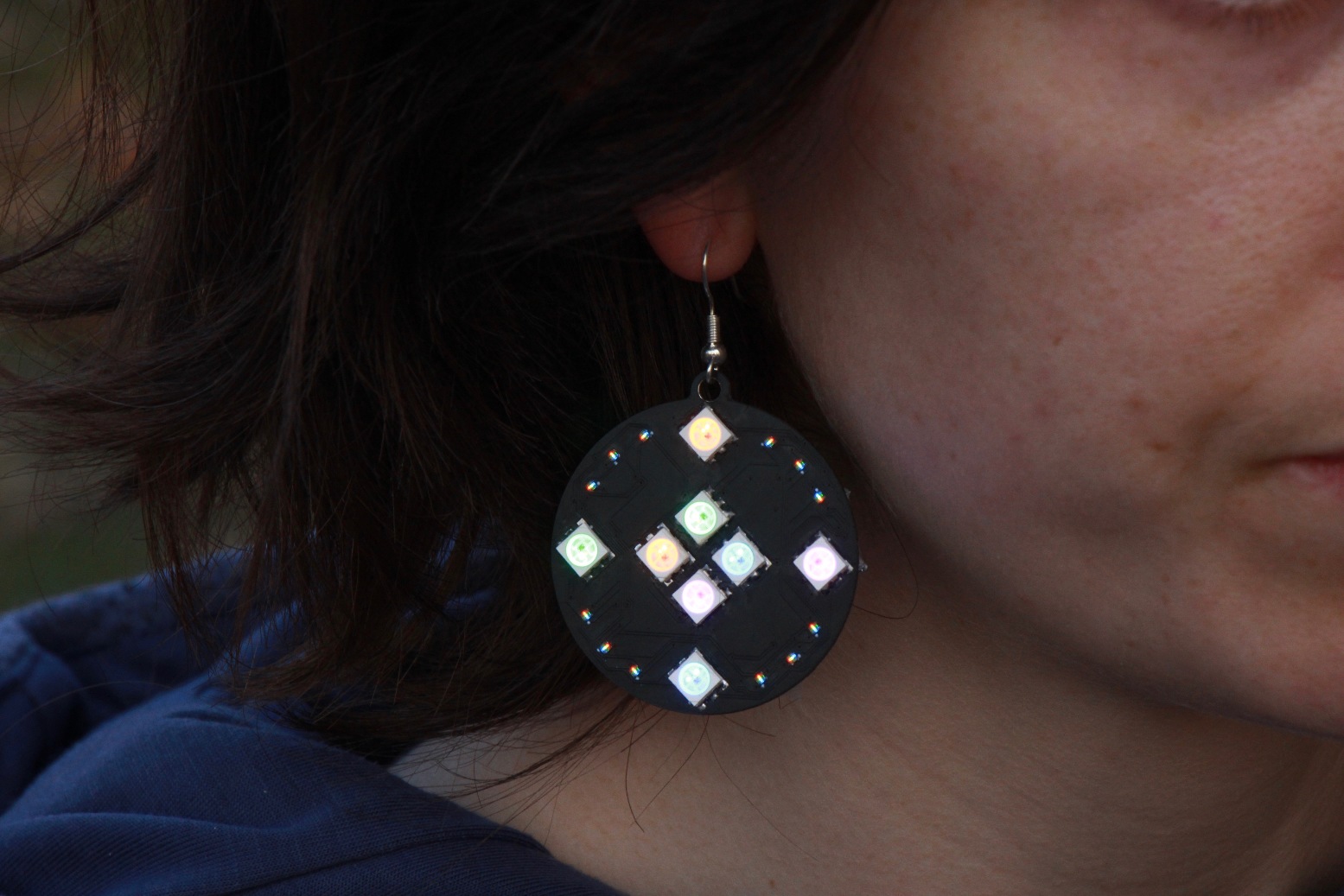
If this piqued your interest, please consider supporting our upcoming Kickstarter. The entirety of the money gathered will be used to organize STEM outreach events for kids, teaching them about Computer Science, Electronics, and Physics. You can sign up to be notified when we launch in March. Or follow the project on twitter @SpinWearables.